You cannot select more than 25 topics
Topics must start with a letter or number, can include dashes ('-') and can be up to 35 characters long.
* Update dependencies * Update module to go 1.17 |
3 years ago | |
---|---|---|
.. | ||
.gitignore | 4 years ago | |
CODE_OF_CONDUCT.md | 4 years ago | |
CONTRIBUTING.md | 4 years ago | |
LICENSE | 4 years ago | |
README.md | 4 years ago | |
build.go | 4 years ago | |
const.go | 4 years ago | |
doc.go | 4 years ago | |
model.go | 4 years ago | |
request.go | 4 years ago | |
resp.go | 4 years ago | |
url.go | 4 years ago |
README.md
Request

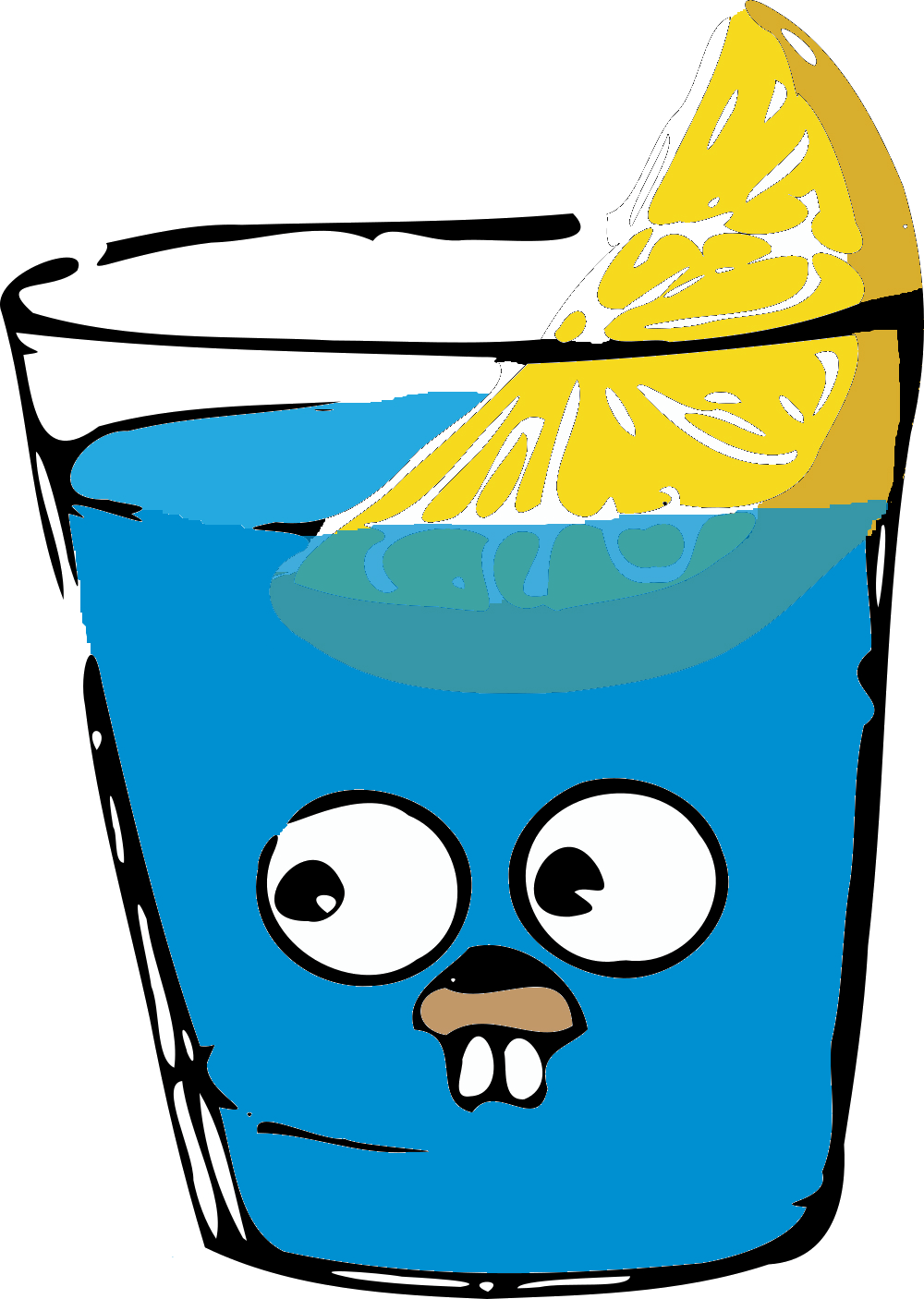
HTTP client for golang, Inspired by Javascript-axios Python-request. If you have experience about axios or requests, you will love it. No 3rd dependency.
Features
- Make http requests from Golang
- Intercept request and response
- Transform request and response data
Installing
go mod:
go get github.com/monaco-io/request
Methods
- OPTIONS
- GET
- HEAD
- POST
- PUT
- DELETE
- TRACE
- CONNECT
Example
GET
package main
import (
"log"
"github.com/monaco-io/request"
)
func main() {
client := request.Client{
URL: "https://google.com",
Method: "GET",
Params: map[string]string{"hello": "world"},
}
resp, err := client.Do()
log.Println(resp.Code, string(resp.Data), err)
}
POST
package main
import (
"log"
"github.com/monaco-io/request"
)
func main() {
client := request.Client{
URL: "https://google.com",
Method: "POST",
Params: map[string]string{"hello": "world"},
Body: []byte(`{"hello": "world"}`),
}
resp, err := client.Do()
log.Println(resp.Code, string(resp.Data), err)
}
Content-Type
package main
import (
"log"
"github.com/monaco-io/request"
)
func main() {
client := request.Client{
URL: "https://google.com",
Method: "POST",
ContentType: request.ApplicationXWwwFormURLEncoded, // default is "application/json"
}
resp, err := client.Do()
log.Println(resp.Code, string(resp.Data), err)
}
Authorization
package main
import (
"log"
"github.com/monaco-io/request"
)
func main() {
client := request.Client{
URL: "https://google.com",
Method: "POST",
BasicAuth: request.BasicAuth{
Username:"user_xxx",
Password:"pwd_xxx",
}, // xxx:xxx
}
resp, err := client.Do()
log.Println(resp.Code, string(resp.Data), err)
}
Timeout
package main
import (
"log"
"github.com/monaco-io/request"
)
func main() {
client := request.Client{
URL: "https://google.com",
Method: "POST",
Timeout: 10, // seconds
}
resp, err := client.Do()
log.Println(resp.Code, string(resp.Data), err)
}
Cookies
package main
import (
"log"
"github.com/monaco-io/request"
)
func main() {
client := request.Client{
URL: "https://google.com",
Cookies:[]*http.Cookie{
{
Name: "cookie_name",
Value: "cookie_value",
},
},
}
resp, err := client.Do()
log.Println(resp.Code, string(resp.Data), err)
}
TLS
package main
import (
"log"
"crypto/tls"
"github.com/monaco-io/request"
)
func main() {
client := request.Client{
URL: "https://google.com",
TLSConfig: &tls.Config{InsecureSkipVerify: true},
}
resp, err := client.Do()
log.Println(resp.Code, string(resp.Data), err)
}